Progress
Mathematics
Started the HELM workbook on Basic Functions. Really nice to see how functions in mathematics and programming are the exact same thing. I liked reading about composing functions.
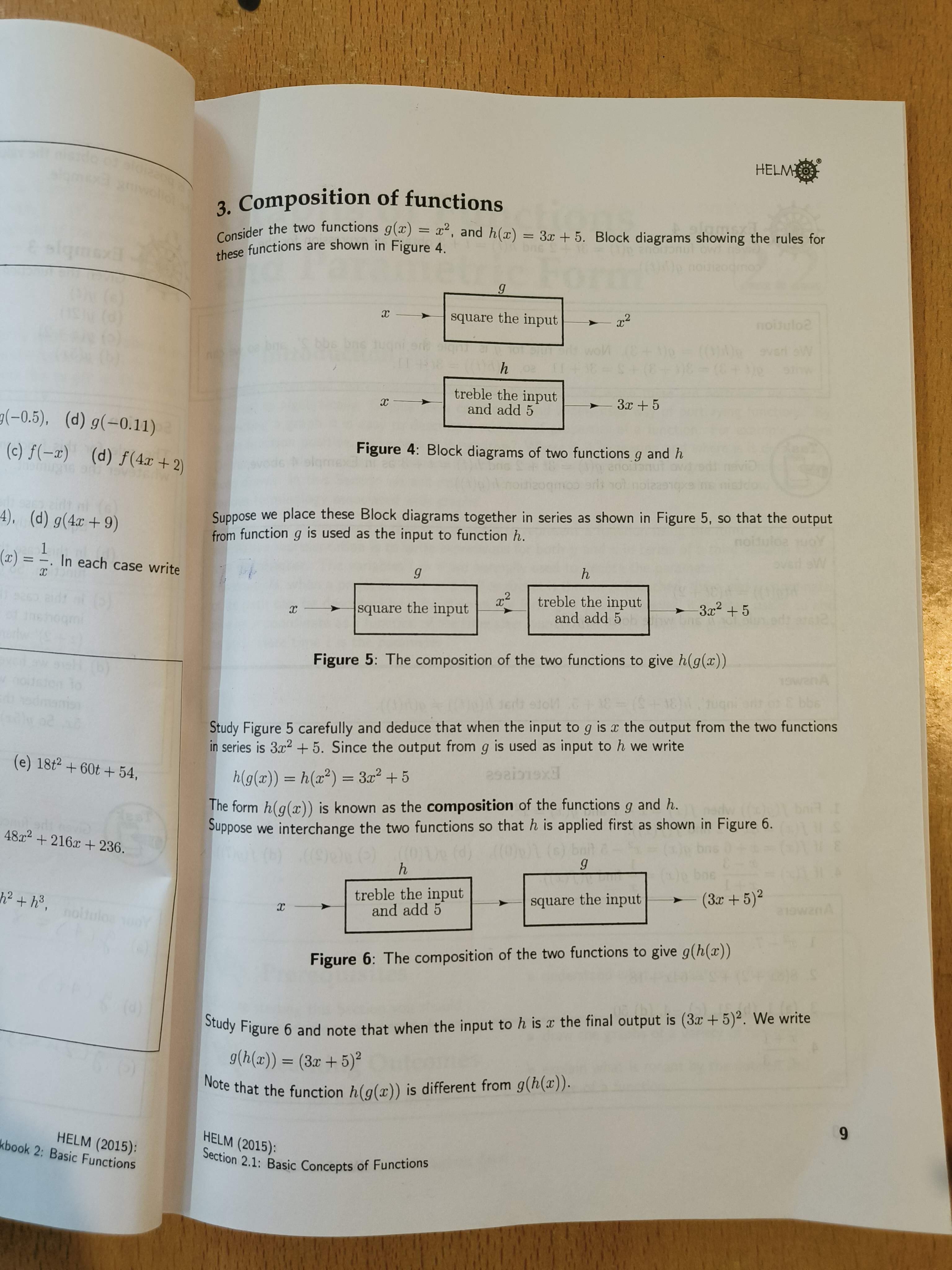
Functional Programming
datatype exp = Constant of int
| Negate of exp
| Add of exp * exp
| Multiply of exp * exp
fun eval e =
case e of
Constant i => i
| Negate e2 => ~ (eval e2)
| Add(e1,e2) => (eval e1) + (eval e2)
| Multiply(e1,e2) => (eval e1) * (eval e2)
val example_exp = Add (Constant 19, Negate (Constant 4))
Evaluating example_exp
results in 15 as we expect.
I love how concise this is. I have done the exact thing in Ruby before. OOP requires creating a class for each data type.
Pleasantly surprised to learn that ML has records! I wrongly assumed that FP languages only have lists and tuples. I never encountered a key value data type when I tried Haskell. Not sure if it does. Most of the discussion was around lists and how amazing they are.